Auro Wallet Integration in React: Complete Guide for Developers
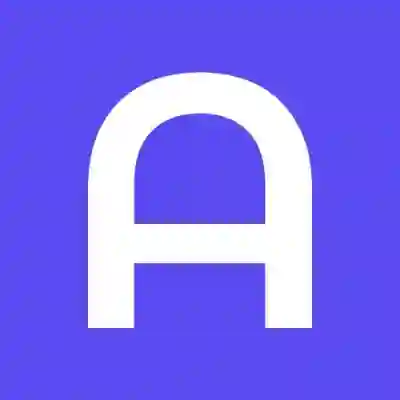
- Authors
- Name
- Alexander Fedotov
- @AlexFedotovqq
- Name
- Pavel Fedotov
- @pfedprog
In this guide, I will tell you about the process of connecting Auro Wallet to your react project. This guide is based on the TradeCoin codebase.
Prerequisites
- Ensure that the Auro Wallet is installed on your device.
Let's get started
In case, you do not have react library installed:
npm install react
This will install the React library into your project.
1. Import Necessary hooks
After that, you can use the hooks by importing them as shown:
import { useEffect, useState } from "react";
2. Define Local Storage Key
const LOCAL_STORAGE_KEY = "MINA";
This key will be used to store the wallet address in the local storage.
connectWallet
Function
3. Implement async function connectWallet(updateDisplayAddress) {
const accounts = await window.mina.requestAccounts();
const displayAddress = `${accounts[0].slice(0, 6)}...${accounts[0].slice(
-4
)}`;
window.localStorage.setItem(
LOCAL_STORAGE_KEY,
JSON.stringify(displayAddress)
);
updateDisplayAddress(displayAddress);
}
This function connects to the Auro Wallet, retrieves the user's address, and updates the displayed address on the TradeCoin website.
getWalletAddress
Function
4. Create function getWalletAddress() {
if (typeof window !== "undefined") {
const value = localStorage.getItem(LOCAL_STORAGE_KEY);
if (value === null) return;
return JSON.parse(value);
}
}
This function retrieves the wallet address from local storage.
WalletButton
Component
5. Define the export const WalletButton = () => {
const [isClient, setIsClient] = useState(false);
const [displayedAddress, updateDisplayAddress] = useState(getWalletAddress());
useEffect(() => {
setIsClient(true);
}, []);
return (
<>
{isClient && (
<div className="flex items-center md:ml-12">
{displayedAddress ? (
<button className="flex w-full items-center justify-center rounded-md border border-transparent bg-indigo-500 px-4 py-3 text-base font-medium text-white hover:bg-indigo-700 md:py-2 md:px-5 ">
{displayedAddress}
</button>
) : (
<button
onClick={() => connectWallet(updateDisplayAddress)}
className="flex w-full items-center justify-center rounded-md border border-transparent bg-indigo-600 px-4 py-3 text-base font-medium text-white hover:bg-indigo-700 md:py-2 md:px-5 "
>
Connect Wallet
</button>
)}
</div>
)}
</>
);
};
This component displays a button that either shows the connected wallet address or prompts the user to connect their wallet.
WalletButton
Component
6. Integrate Place the WalletButton
component where you want the wallet connection button to appear on your website. For example:
import { WalletButton } from "./path/to/WalletButton";
function App() {
return (
<div>
{/* Other components */}
<WalletButton />
</div>
);
}
7. Customize Styling
Adjust the button styling in the WalletButton
component according to your website's design.
8. Test the Integration
Open your website in a browser, ensure that the Auro Wallet is installed, and click the Connect Wallet button. Verify that the wallet address is displayed upon successful connection.
- Step
1
- Step
2
- Step
3
That's it! You have successfully integrated the Auro Wallet connection function
Related Links and Posts
- Demystifying the Filecoin Virtual Machine (FVM)
- Simple App with Ceramic Data Model and Unstoppable Domains
- Empowering DeFi with Synthetic Assets
- The Optimism Ecosystem and RetroPGF: Nurturing Innovation and Impact
- Unlock the Secrets of Zero Knowledge Technology with Lauri Peltonen
- How to illustrate log returns vs simple returns
- A How to EfficientNet Classification
- Cross-sectional data – An easy introduction
- Zero Knowledge proofs on Mina, zkPassport and SoulBound NFTs